An Introduction to Web Development with PLT Scheme - The View
Introduction
First of all, thanks for the positive response to part 1 of this introduction. Comments are very welcome.
The goal of this introduction is to write a "Mini Reddit" called "List It!". The first part focused on the model component i.e. the underlying data and how to implement it using an SQLite database. This second part will concentrate on the view component, which presents the data to the user. The third part will be on the controller.
Our user interface consists of two pages: the frontpage shows the list of links with the highest scores and the submission page where a user can enter the name and url of a new entry. To give an impression of where we are headed, here is screenshots of the final result:
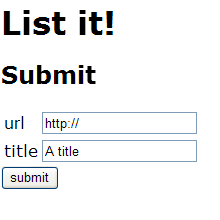
In the Model-View-Controller organization, the controller receives a request from the client (user), retrieves data from the model, lets the view construct a web page and the sends a response back to the client. The view thus consists of a series of functions turning data
into web-pages.
Representing XHTML as X-expressions
PLT Scheme supports multiple ways of representing web-pages. One option is to use strings:
Instead we will use X-expressions (S-expressions representing XML) to represent our web-pages. The above example becomes:
The beauty of X-expressions is that, since they are normal lists, we can use the builtin functions to manipulate them. Another major convenience is we can use unquote (written as a comma) to insert the result of a Scheme expressions into the X-expression:
To avoid writting boilerplate stuff (charset, stylesheet, content type etc.) each and every time a web page is constructed, we will use the general web framework from PLaneT (the PLT Scheme "CPAN" - note that packages are downloaded automatically if needed).
In the example above, the html-page only received the body, so it used the site wide default arguments for the title, the stylesheet and the "header". Obviously we need to override the defaults for our List It! site:
After overriding the defaults we get:
Use the keywords #:title, #:header, #:body, #:style-sheet, and #:style-sheet with html-page, in order not to use the site wide defaults.
The Submit-new-entry page
The funtion html-submit-new-page builds an X-expression representing the submission page. It takes no arguments, since it doesn't rely on data from the model:
The submission page consists of a form with two input fields. One for the url and one for the title. The function html-form rececives a name of the form, the action. The default method for submitting is POST, but one can use the keyword argument #:method to choose something else. The function html-input builds an input field. Other types of input fields are: 'image and 'hidden.
Clicking the submit button will post the parameters action, url and title to control.scm.
The Frontpage
The submitted entries are ranked according to their score. The entry with the highest score has rank 1, the next rank 2 and so on. Only a limited number of entries can be showed at a time, so we imagine the entries divided into pages. The function html-front-page below receives three arguments: a page number, the rank of the first entry of the page in question and the list of entries
The function html-menu generates an X-expression for the "menu" on top of the screen The function html-list-of-entries generates an X-expression representing the list of entries.
The menu
The "menu" consists so far of a single menu item "submit-new-link".
The list of entries
The list of entries is received by html-list-of-entries as a list of vectors.
The first vector holds the column names in the database, and can thus safely be ignored.
A DIV of class "entries" is used around the list of entries, so we single them out in the stylesheet. Next we use an eager comprehension list-ec to generate a list of X-expressions, one for each entry. Since we want the result to be of the form (div (class "entries") (table ...) (table ...) ...) and not (div (class "entries") ((table ...) (table ...) ...)) we use unquote-splicing ,@ instead of just unquote.
The eager comprehension first checks to see, whether entries is the empty list - and if so, an empty list of X-expressions is generated.
The clause (list entry (index i) (cdr entries)) iterates over the elements of the list entries (minus the first element), binding entry to each element in turn, also the index variable i counts from 0 and up.
The clause (:match #(id header url score) entry) uses pattern matching to bind the variables id, header, url, and score to the elements of the entry vector.
The final expression `(table ...) can now use the variables to generate the X-expression for the entry in question.
The table is used to position the rank, the up- and down-arrow and the link. I am able to do the same in CSS - but not in all browsers at the same time.
The alert reader will probably wonder, why the form is introduced. Why aren't the arrows two simple links to, say,
control.scm?action=updown&arrowitem=down&entry_id=1 ?
Well, a click at the above url would result in the entry getting a vote. The problem lies in what happens next. If the user now reloads the page, the entry will get an extra vote. Bookingmarking the page will also result in extra votes, when the user returns.
The form-solution sends the parameters "behind the scenes", so that the url becomes control.scm. This obviously solves the bookmarking problem. The reload problem is solved via the Post/Redirect/Get pattern - which I'll discuss in detail in the post on the controller.
The function html-a-submit builds a link that submits the form (it's in the web-framework), it involves a little JavaScript.
The function html-icon returns an X-expression `(img ...) representing various images. In this tutorial only the up- and down-arrow is supported, but if anyone is interested, I have a version supporting all of Tango (an excellent source of icons).
The Program
First of all, thanks for the positive response to part 1 of this introduction. Comments are very welcome.
The goal of this introduction is to write a "Mini Reddit" called "List It!". The first part focused on the model component i.e. the underlying data and how to implement it using an SQLite database. This second part will concentrate on the view component, which presents the data to the user. The third part will be on the controller.
Our user interface consists of two pages: the frontpage shows the list of links with the highest scores and the submission page where a user can enter the name and url of a new entry. To give an impression of where we are headed, here is screenshots of the final result:
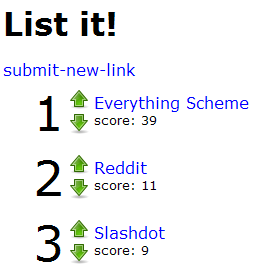
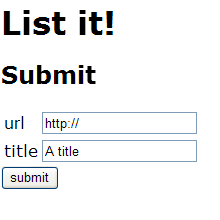
In the Model-View-Controller organization, the controller receives a request from the client (user), retrieves data from the model, lets the view construct a web page and the sends a response back to the client. The view thus consists of a series of functions turning data
into web-pages.
Representing XHTML as X-expressions
PLT Scheme supports multiple ways of representing web-pages. One option is to use strings:
Since the structure of the document is lost with this choice, all but the most simple manipulations of the document, becomes harder to program than they should.
"<html><head><title>A Title</title>
<body>A body</body></html>".
Instead we will use X-expressions (S-expressions representing XML) to represent our web-pages. The above example becomes:
`(html (head (title "A title"))Besides X-expressions another popular choice in the Scheme world is SXML (if you are into serious XML-manipulations look into SXML and SSAX), which are similar to X-expressions in terms of use.
(body "A body"))
The beauty of X-expressions is that, since they are normal lists, we can use the builtin functions to manipulate them. Another major convenience is we can use unquote (written as a comma) to insert the result of a Scheme expressions into the X-expression:
`(body "The result of 1+2 is: " ,(number->string (+ 1 2)))which, when evaluated, results in
(body "The result of 1+2 is: " "3").Attributes are placed right after the tag:
`(body ((color "white") (bgcolor "black"))The WEB library
"Hello world")
To avoid writting boilerplate stuff (charset, stylesheet, content type etc.) each and every time a web page is constructed, we will use the general web framework from PLaneT (the PLT Scheme "CPAN" - note that packages are downloaded automatically if needed).
> (require (planet "html.scm" ("soegaard" "web.plt" 1 0)))
> (html-page #:body `(p "Hello world"))
(html
(head (title "A title")
(link ((rel "stylesheet") (type "text/css") (href "")))
(meta ((http-equiv "Content-Type") (content "text/html;charset=UTF-8"))))
(body (h1 "A Header")
(p "Hello world")))
(override-default current-page-title"List it!")
(override-default current-page-header'(h1 ((class "page_header")) "List it!"))
(override-default current-page-style-sheet "http://localhost/stylesheet.css")
After overriding the defaults we get:
(html
(head (title "List it!")
(link ((rel "stylesheet") (type "text/css")
(href "http://localhost/stylesheet.css")))
(meta ((http-equiv "Content-Type")
(content "text/html;charset=UTF-8"))))
(body (h1 ((class "page_header")) "List it!")
(p "Hello world")))
Use the keywords #:title, #:header, #:body, #:style-sheet, and #:style-sheet with html-page, in order not to use the site wide defaults.
The Submit-new-entry page
The funtion html-submit-new-page builds an X-expression representing the submission page. It takes no arguments, since it doesn't rely on data from the model:
(define (html-submit-new-page)
(html-page
#:title "List it! - submit"
#:header '(h1 "List it!")
#:body
`(div (h2 "Submit a new entry")
,(html-form
"submitnewform" "control.scm"
(html-input "action" #:value "submit" #:type 'hidden)
`(table (tr (td "url") (td ,(html-input "url" #:type 'text #:value "http://")))
(tr (td "title") (td ,(html-input "title" #:type 'text #:value "A title"))))
(html-input "submit" #:value "submit")))))
The submission page consists of a form with two input fields. One for the url and one for the title. The function html-form rececives a name of the form, the action. The default method for submitting is POST, but one can use the keyword argument #:method to choose something else. The function html-input builds an input field. Other types of input fields are: 'image and 'hidden.
Clicking the submit button will post the parameters action, url and title to control.scm.
The Frontpage
The submitted entries are ranked according to their score. The entry with the highest score has rank 1, the next rank 2 and so on. Only a limited number of entries can be showed at a time, so we imagine the entries divided into pages. The function html-front-page below receives three arguments: a page number, the rank of the first entry of the page in question and the list of entries
(define (html-front-page page-number rank-of-first-entry entries)
(html-page
#:body `(div
,(html-menu)
,(html-list-of-entries page-number rank-of-first-entry entries))))
The menu
The "menu" consists so far of a single menu item "submit-new-link".
(define (html-menu)
`(a ((href "control.scm?action=submitnew")) "submit-new-link"))
The list of entries is received by html-list-of-entries as a list of vectors.
(#4("entry_id" "title" "url" "score")
#4("1" "Everything Scheme" "http://www.scheme.dk/blog/" "42")
#4("3" "PLT Scheme" "http://www.plt-scheme.org" "9")
...)
The first vector holds the column names in the database, and can thus safely be ignored.
(define (html-list-of-entries page-number rank-of-first-entry entries)
`(div ((class "entries"))
,@(list-ec
(if (not (null? entries)))
(:list entry (index i) (cdr entries))
(:match #(id header url score) entry)
`(table ...))))
A DIV of class "entries" is used around the list of entries, so we single them out in the stylesheet. Next we use an eager comprehension list-ec to generate a list of X-expressions, one for each entry. Since we want the result to be of the form (div (class "entries") (table ...) (table ...) ...) and not (div (class "entries") ((table ...) (table ...) ...)) we use unquote-splicing ,@ instead of just unquote.
The eager comprehension first checks to see, whether entries is the empty list - and if so, an empty list of X-expressions is generated.
The clause (list entry (index i) (cdr entries)) iterates over the elements of the list entries (minus the first element), binding entry to each element in turn, also the index variable i counts from 0 and up.
The clause (:match #(id header url score) entry) uses pattern matching to bind the variables id, header, url, and score to the elements of the entry vector.
The final expression `(table ...) can now use the variables to generate the X-expression for the entry in question.
The table is used to position the rank, the up- and down-arrow and the link. I am able to do the same in CSS - but not in all browsers at the same time.
`(table ((class "entry"))
(tr (td ((class "rank")) ,(number->string (+ i rank-of-first-entry)))
(td ,(let ((form (format "arrowform~a" id)))
(html-form form "control.scm"
#:atts '((class "arrows"))
(html-input "arrowitem" #:type 'hidden)
(html-input "entry_id" #:type 'hidden #:value id)
(html-input "action" #:type 'hidden #:value "updown")
`(div ,(html-a-submit form "arrowitem" "up"
(html-icon 'go-up #:class "arrow")))
,(html-a-submit form "arrowitem" "down"
(html-icon 'go-down #:class "arrow")))))
(td (div (a ((href ,url)) ,header))
(span ((class "score")) "score: " ,score)))))))
control.scm?action=updown&arrowitem=down&entry_id=1 ?
Well, a click at the above url would result in the entry getting a vote. The problem lies in what happens next. If the user now reloads the page, the entry will get an extra vote. Bookingmarking the page will also result in extra votes, when the user returns.
The form-solution sends the parameters "behind the scenes", so that the url becomes control.scm. This obviously solves the bookmarking problem. The reload problem is solved via the Post/Redirect/Get pattern - which I'll discuss in detail in the post on the controller.
The function html-a-submit builds a link that submits the form (it's in the web-framework), it involves a little JavaScript.
(define (html-a-submit formname formitem id text)
`(a ((href ,(string-append
(format "javascript:document.~a.~a.value='~a';" formname formitem id)
(format "document.~a.submit();" formname))))
,text))
The Program
;;; view.scm -- Jens Axel Soegaard
(module view mzscheme
(provide (all-defined))
(require (lib "kw.ss")
(planet "42.ss" ("soegaard" "srfi.plt"))
(planet "html.scm" ("soegaard" "web.plt" 1 0))
(planet "web.scm" ("soegaard" "web.plt" 1 0)))
;;;
;;; SITE WIDE DEFAULTS
;;;
(override-default current-page-title "List it!")
(override-default current-page-header '(h1 ((class "page_header")) "List it!"))
(override-default current-page-style-sheet "http://localhost/stylesheet.css")
;;;
;;; FRONT PAGE(S)
;;;
(define (html-front-page page-number rank-of-first-entry entries)
(html-page
#:body `(div
,(html-menu)
,(html-list-of-entries page-number rank-of-first-entry entries))))
(define (html-menu)
`(a ((href "control.scm?action=submitnew")) "submit-new-link"))
(define (html-submit-new-page)
(html-page
#:title "List it! - submit"
#:header '(h1 "List it!")
#:body
`(div (h2 "Submit a new entry")
,(html-form
"submitnewform" "control.scm"
(html-input "action" #:value "submit" #:type 'hidden)
`(table (tr (td "url") (td ,(html-input "url" #:type 'text #:value "http://")))
(tr (td "title") (td ,(html-input "title" #:type 'text #:value "A title"))))
(html-input "submit" #:value "submit")))))
(define/kw (html-icon name #:key (class #f))
(define (icon-absolute-url name)
(format "/~a.png" name))
(if class
`(img ((class ,class) (src ,(icon-absolute-url name))))
`(img ( (src ,(icon-absolute-url name))))))
(define (html-list-of-entries page-number rank-of-first-entry entries)
`(div ((class "entries"))
,@(list-ec
(if (not (null? entries)))
(:list entry (index i) (cdr entries))
(:match #(id header url score) entry)
`(table ((class "entry"))
(tr (td ((class "rank")) ,(number->string (+ i rank-of-first-entry)))
(td ,(let ((form (format "arrowform~a" id)))
(html-form form "control.scm"
#:atts '((class "arrows"))
(html-input "arrowitem" #:type 'hidden)
(html-input "entry_id" #:type 'hidden #:value id)
(html-input "action" #:type 'hidden #:value "updown")
`(div ,(html-a-submit form "arrowitem" "up" (html-icon 'go-up #:class "arrow")))
(html-a-submit form "arrowitem" "down" (html-icon 'go-down #:class "arrow")))))
(td (div (a ((href ,url)) ,header))
(span ((class "score")) "score: " ,score)))))))
; html-redirect-page
; a standard text to show, when redirecting
(define (html-redirect-page body)
(html-page #:title "Redirecting"
#:body body))
)
8 Comments:
Thank you for this tutorial. As a newcommer to Scheme, this is a great and inspiring way to get started, and I'm already having a go at making a simple wiki by using what I've learned so far.
I am very much looking forward to the last part of this example.
That's great news. I'd love to try it some day.
thanks for this post!
When can we expect the 3rd part?
Great series. Are you planning on a third anytime soon?
Hi Ido and Pragmatic,
I've been busy at work, but the part "2.5" is now available at
An Introduction wot Web Development with PLT Scheme - An Intermezzo.
two simple, helpful scripts when actively developing with plt server on linux.
1) script named "refserv"
wget "http://localhost:8000/conf/refresh-servlets"
rm refresh-servlets
2) script named "looprefserv"
while true; do refserv; sleep 20; done
3) either run "refserv &" or "looprefserv &"
I am trying to learn plt web server..
How to print all the header information on html page..
like all the request parameters..
which function to use...
Hi gangadhar,
Maybe you can use something from the
Net documentation?
Post a Comment
Links to this post:
Create a Link
<< Home